C++
Computational Graphics
This project in computational graphics was completed using OpenGL in C++. While it was fun to learn how to recreate pictures into late ‘90s era graphics, my real takeaway from this project was taking an existing, and initially intimidating codebase, breaking it down, understanding it, then using it to finish my project.
The main skills this exemplifies are:
- Being able to understand and operate in C++.
- Learning new skills quickly, then being able to translate those skills into real world results.
- Taking an existing codebase and being able to break it down into its component parts to then add to and manipulate it.
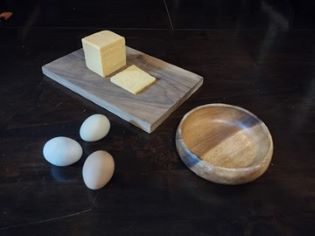
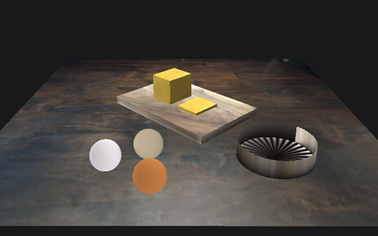
Code files:
Data Structures and Algorithms
My data structures and algorithms projects were in C++ as well. Like the computational graphics, this doesn’t show any great skill in C++, but does show I can take a task, understand the fundamentals, and execute the code in C++.
Sample from the binary search tree project:
Node* BinarySearchTree::removeNode(Node* node, string bidId) {
if (node == nullptr) {
return node;
}
// If bidId of node is greater than bidId to remove, go to left branch
if (node->bid.bidId.compare(bidId) > 0) {
node->left = removeNode(node->left, bidId);
}
// If bidid of node is less than bidId to remove, go to right branch
else if (node->bid.bidId.compare(bidId) < 0) {
node->right = removeNode(node->right, bidId);
}
// Current node contains bid to remove
else {
// If node has no children, delete node
if (node->left == nullptr && node->right == nullptr) {
delete node;
node = nullptr;
}
// If node has one left child, node = left node, then delete node
else if (node->left != nullptr && node->right == nullptr) {
Node* temp = node;
node = node->left;
delete temp;
}
// If node has one right child, node = right, then delete node
else if (node->left == nullptr && node->right != nullptr) {
Node* temp = node;
node = node->right;
delete temp;
}
// If node has two children, find the leftmost node of the right child
// Make that node's bid the current node's bid, then remove that node
else {
Node* temp = node->right;
while (temp->left != nullptr) {
temp = temp->left;
}
node->bid = temp->bid;
node->right = removeNode(node->right, temp->bid.bidId);
}
}
return node;
}